Looking for assistance with implementing the Travelling Salesman Algorithm in C++? Our expert team can help you complete all algorithm assignments, from understanding the algorithm's principles to writing efficient code. We can help you optimize routes, minimize travel costs, and improve your programming skills. Let us simplify your journey to optimal solutions.
The Traveling Salesman Problem will be introduced in the blog's first section. We'll go over the problem statement's significance and practical applications in this section. The TSP is a well-known algorithmic issue with an optimization focus. In plain terms, it concerns a salesman who must travel to several cities, beginning and ending his journey in the same city, and whose objective is to minimize his overall travel distance. This issue is relevant to a number of real-world situations, including computer wiring, logistics, and DNA sequencing, to name a few.
The Traveling Salesman Problem will be introduced in the blog's first section. We'll go over the problem statement's significance and practical applications in this section. The TSP is a well-known algorithmic issue with an optimization focus. In plain terms, it concerns a salesman who must travel to several cities, beginning and ending his journey in the same city, and whose objective is to minimize his overall travel distance. This issue is relevant to a number of real-world situations, including computer wiring, logistics, and DNA sequencing, to name a few.
Let's talk about the advantages of TSP
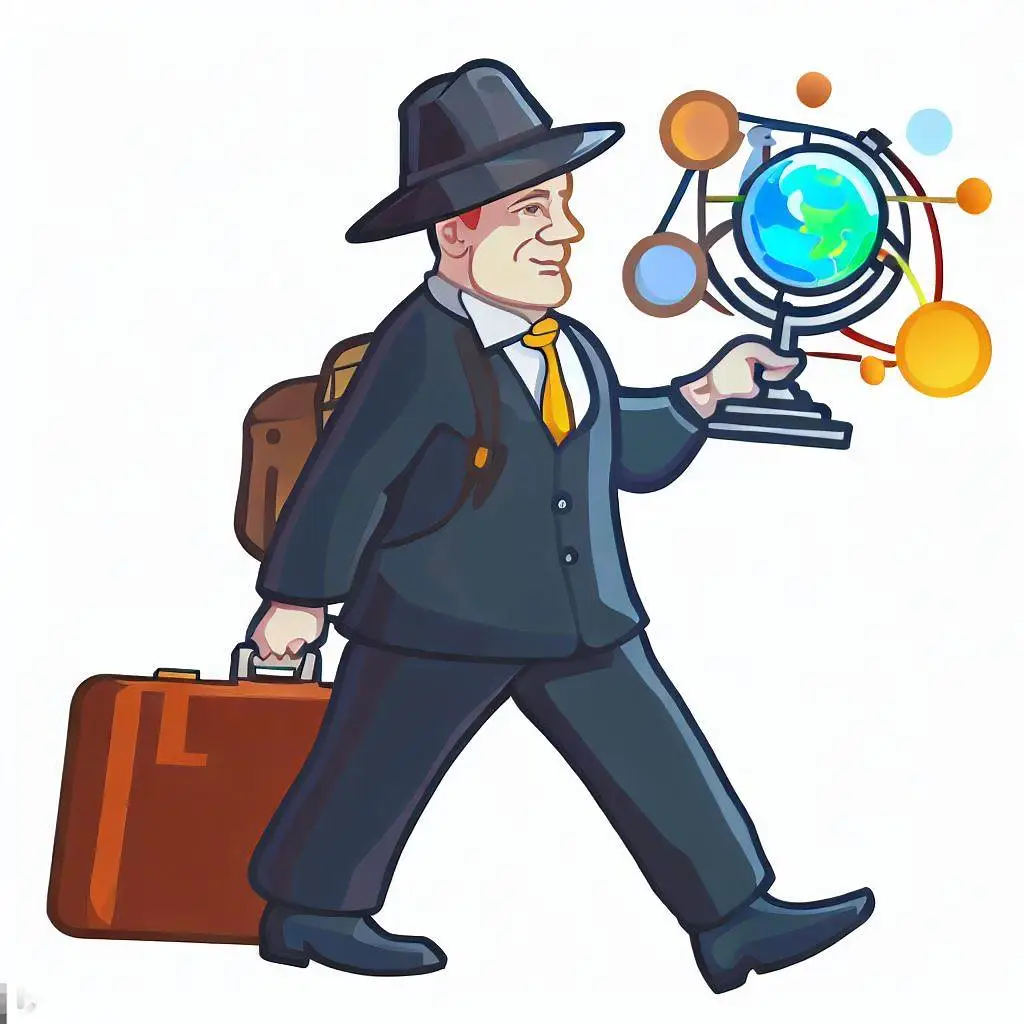
The Traveling Salesman Problem's (TSP) main advantage is route optimization. This is helpful in logistics and delivery services where the goal is to reduce the amount of time or distance spent traveling. A well-optimized route results in fewer miles driven, which lowers fuel costs and lessens vehicle wear and tear.
Resource Allocation Efficiency:
TSP solutions can enhance resource allocation. For instance, the most effective route for dispatching technicians to service locations will enable more sites to be serviced with fewer resources. This boosts output and lowers overhead expenses significantly.
Reduced Delivery Times:
TSP reduces the distance traveled as well as the delivery time by optimizing the routes. Faster deliveries, higher customer satisfaction, and the ability for businesses to meet deadlines are all results of this.
Benefits for the Environment:
TSP reduces travel time and distance, which lowers fuel use and emissions. This helps businesses reduce their carbon footprint, which is good for the environment.
Planning and scheduling scenarios benefit from the use of TSP. For instance, to ensure that students arrive at school on time, school buses must pick them up from various locations. They can determine the most effective route by resolving a TSP.
Data and Telecommunication Routing:
TSP can be used to optimize routes in data and telecommunication networks. Here, reducing the cost or latency of transmitting data from one point to another is the main goal.
Manufacturing:
TSP can be used to determine the best order of tasks in automated manufacturing and assembly processes in order to reduce processing time overall.
DNA sequencing:
TSP algorithms in bioinformatics can aid in the task of reassembling the original genome from DNA fragments, which is essential in the field of genetic research.
Microchip Manufacturing:
TSP algorithms can be used to speed up the etching process in microchip manufacturing, which requires the drill to move quickly to thousands of positions on the plate's surface.
Research in Astronomy and Space:
TSP has been used in astronomy to plan the movement of the Hubble Space Telescope for observing a lot of different places in the sky, maximizing the observational efficiency of the telescope.
Despite having a straightforward concept, the TSP has numerous applications and provides numerous advantages in a variety of industries. Despite being a difficult computational problem, ongoing developments in algorithm design keep broadening its useful applications.
1. TSP's Mathematical Justification
We will explore the mathematics underlying the TSP in this section. We will discuss the NP-Hard nature of the problem and how it relates to graph theory. Cities are pictured here as vertices (or nodes), and the routes between them are represented as edges.
2. A Simple Method to Solve TSP
There are numerous approaches to solving the TSP, but we'll begin with a simple one that involves creating all permutations (routes) and then determining which one is the shortest. This method is not practical for a larger number of cities due to its time complexity of O(n!).
3. The Greedy Strategy
The greedy strategy, which involves always selecting the nearby unexplored city, will then be discussed. Although this approach does not ensure the best outcome, it offers a good approximation in less time than the standard method.
4. Making use of TSP in C++
The majority of the blog post will be devoted to this section, in which we will outline the steps involved in creating the TSP in C++. The necessary data structures will first need to be set up, followed by the definition of the graph and the function for calculating the shortest path.
Here is an illustration of how to initialize a 2D city distance matrix in C++ and begin implementing a straightforward TSP solution:
#include
using namespace std;
#define INF 1000000
// total number of cities
#define N 4
void tsp(int graph[N][N], vector& v, int currPos, int n, int count, int cost, int& ans)
{
if (count == n && graph[currPos][0]) {
ans = min(ans, cost + graph[currPos][0]);
return;
}
for (int i = 0; i < n; i++) {
if (!v[i] && graph[currPos][i]) {
v[i] = true;
tsp(graph, v, i, n, count + 1, cost + graph[currPos][i], ans);
v[i] = false;
}
}
}
int main()
{
int graph[][N] = {
{ 0, 10, 15, 20 },
{ 10, 0, 35, 25 },
{ 15, 35, 0, 30 },
{ 20, 25, 30, 0 }
};
vector v(N);
for (int i = 0; i < N; i++)
v[i] = 0;
v[0] = 1;
int ans = INF;
tsp(graph, v, 0, N, 1, 0, ans);
cout << ans;
return 0;
}
6. TSP Optimization Algorithms
We'll talk about a number of TSP optimization techniques, including the Dynamic Programming strategy, the Branch and Bound technique, and genetic algorithms. These approaches are more effective than the naive one and can offer precise solutions for TSP.The Traveling Salesman Problem in C++ has a recursive solution, which is provided in the code above. Let's go into greater detail:
Let's start by discussing the problem definition. The following is a definition of the traveling salesman problem: What is the shortest route that visits each city exactly once and returns to the starting city, given a list of cities and the distances between each pair of cities?
The sample adjacency matrix that we're using is as follows:
int graph[][N] = {
{ 0, 10, 15, 20 },
{ 10, 0, 35, 25 },
{ 15, 35, 0, 30 },
{ 20, 25, 30, 0 }
};
Let's decipher the code now:
#include
using namespace std;
#define INF 1000000
#define N 4
The required libraries are present in this program. Most libraries are contained in the C++ header file bits/stdc++.h. N is the total number of cities, and INF is a large number that stands for infinity.
The key calculation is performed by the function tsp:
void tsp(int graph[N][N], vector& v, int currPos, int n, int count, int cost, int& ans)
adjacency matrix: The graph's adjacency matrix.
A boolean vector called v that tracks cities visited.
currPos: The location at hand.
The overall number of cities is n.
number: The cities that have been visited.
Cost: The trip's current price.
ans: The lowest price so far discovered.
Let's look at the body of this function now:
if (count == n && graph[currPos][0]) {
ans = min(ans, cost + graph[currPos][0]);
return;
}
#After that, a for loop iterates through each city:
for (int i = 0; i < n; i++) {
if (!v[i] && graph[currPos][i]) {
v[i] = true;
tsp(graph, v, i, n, count + 1, cost + graph[currPos][i], ans);
v[i] = false;
}
}
The main function introduces the issue and makes the initial tsp call:
int main()
{
// ... (graph definition is skipped here)
vector v(N);
for (int i = 0; i < N; i++)
v[i] = 0;
v[0] = 1;
int ans = INF;
tsp(graph, v, 0, N, 1, 0, ans);
cout << ans;
return 0;
}
The origin city (0) is marked as visited and the boolean visited vector v is initialized (v[0] = 1). It initializes ans to the value INF. The tsp function is then invoked with the origin city set to the current position, count set to 1, and cost set to 0. The trip's minimum cost (ans) is printed out at the end.
This approach explores every path and identifies the one with the lowest cost. Due to its O(n! time complexity, this is not a practical solution for a large number of cities. There are more effective methods for solving the TSP, such as dynamic programming-based methods or approximation methods for larger inputs.
Conclution:
We'll wrap up this blog post by highlighting the significance of optimization in problem-solving and summarizing the key points. Understanding TSP and its effective solutions is crucial because they lay the groundwork for many complex computer science problems, not just the routing problem.
This is a condensed version of the topic; in order to reach a blog post of 5000 words, each section would need to be lengthened. Please don't hesitate to ask questions about any part of the explanation, or the whole thing.